How To Draw Touch Screen Buttons In Arduino
We've done quite a number of tutorials on the utilise of several displays with Arduino boards and today we volition add another tutorial to that list. We will look at theILI9325 based two.8″ touchscreen display shown below and how it can be used with the Arduino to deliver a amend user feel for your projects.
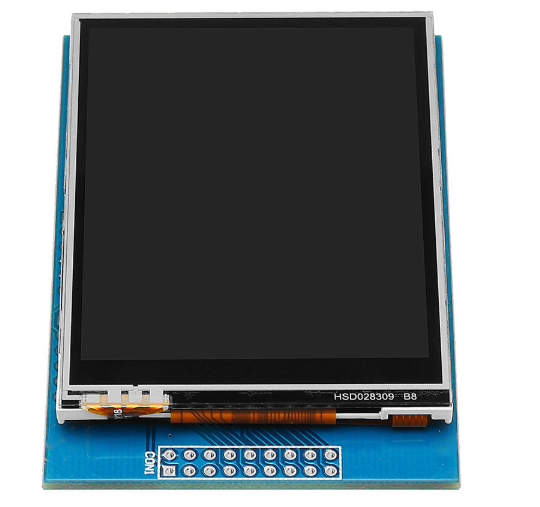
For today's tutorial, nosotros will employ the ILI9325 commuter based, 2.8″ display from Geekcreit. The display comes every bit a shield so it's ready to be used for Arduino based projects. It is an xviii-scrap color display with a total of 262,000 different color shades. The display has a resolution of 240 x 320 pixels with private pixel command.
Today's project involves some very simple tasks which we volition utilise to demonstrate the capabilities of the display. Nosotros will create a button which when touched, volition trigger the Arduino to display a message on the screen. At the end of today'due south tutorial, we would have gone through how to create a user interface on the touchscreen, how to detect when the screen is touched and how to display data on the screen.
Required Components
The following components/parts are required to build this projection:
- 2.8″ Affect Screen
- Arduino Uno
- Arduino Mega
- Xiaomi Powerbank
The Arduino Mega or whatever of the other Arduino board tin be used for this project and the power banking concern comes in handy when the project is to be used in a standalone style. As usual, the exact components used for this tutorial can be purchased via the links attached to each of them.
Schematics
The 2.viii″ TFT brandish used for this project comes equally a shield with the form factor of the Arduino Uno. This makes it piece of cake to connect the shield to boards like the Uno, Mega and Due, as all we need to do, is plug information technology directly into the board, eliminating all the mess made by wires. Plug the display to the Arduino every bit shown in the image below.
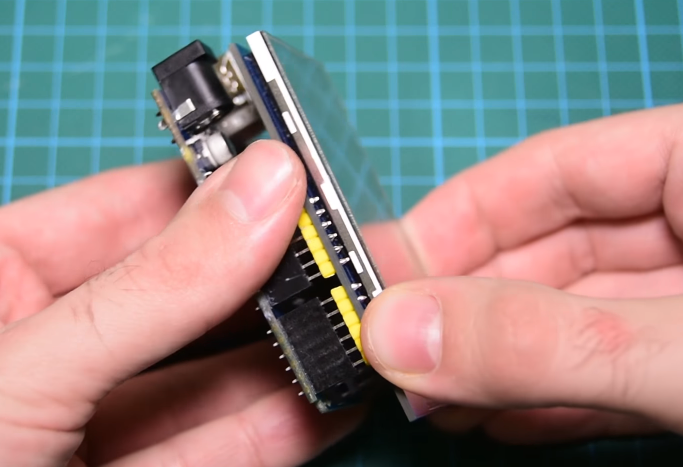
The fact that the display comes every bit a shield becomes a disadvantage when its used with the Arduino Uno as it occupies almost all the pins leaving but 2 digital pins and one analog pin for other uses. This can however, be overcome by using either the Arduino Mega or Due every bit they both work perfectly well with the display.
Code
The code for this tutorial is heavily reliant on a modified version of Adafruit's TFT LCD, GFX and touchscreen libraries. These libraries tin can exist downloaded from the links attached to them.
As mentioned earlier, our focus for this tutorial will be to demonstrate, how UI tin be created on the display and interpret touches to trigger actions. To achieve this, we volition develop a simple sketch which displays a Youtube subscribe push. When the subscribe button is pressed, a text is displayed on the screen.
To do a quick run through the code, we volition start by including the libraries that will be used for the project.
//Written by Nick Koumaris // info@educ8s.boob tube #include <Adafruit_TFTLCD.h> #include <Adafruit_GFX.h> #include <TouchScreen.h>
Adjacent, we declare the pins of the Arduino to which the display is connected.
#define LCD_CS A3 #define LCD_CD A2 #define LCD_WR A1 #define LCD_RD A0 #ascertain LCD_RESET A4
Calibration needs to be washed before the touchscreen functionality of this display tin can exist used. To calibrate the screen, nosotros upload the code and Open the Serial Monitor to obtain the values of the display's edges. Click (touch on) on the superlative left corner of the display and write down the X and Y values displayed on the serial monitor. Then nosotros edit the code to reflect those values. The X value goes to the TS_MAXX variable and the Y value goes to the TS_MAXY variable. Side by side, click on the bottom right corner of the display and enter the values displayed on the serial monitor for the TS_MINX and TS_MINY variables. With this done our display is now calibrated and ready for use.
#ascertain TS_MINX 204 #define TS_MINY 195 #define TS_MAXX 948 #ascertain TS_MAXY 910
Next, nosotros declare the colors to be used with their hexadecimal values and we create an object of the Adafruit TFTLCD library, indicating the variables used to represent the pins of the Arduino to which the display is continued.
#define BLACK 0x0000 #define Blueish 0x001F #define Scarlet 0xF800 #ascertain Green 0x07E0 #ascertain CYAN 0x07FF #define MAGENTA 0xF81F #define Yellowish 0xFFE0 #define WHITE 0xFFFF Adafruit_TFTLCD tft(LCD_CS, LCD_CD, LCD_WR, LCD_RD, LCD_RESET);
We also create an object of the touchscreen library indicating, the pins of the Arduino to which, the impact part of the screen is connected.
TouchScreen ts = TouchScreen(XP, YP, XM, YM, 300);
next, we write the Setup function.
We start past initializing the serial monitor and the display. After this, we gear up the orientation of the LCD and fill the screen with a black color to serve as the background.
void setup() { Serial.brainstorm(9600); Serial.print("Starting..."); tft.reset(); tft.begin(0x9325); tft.setRotation(ane); tft.fillScreen(BLACK);
next, we draw a white frame on the display, set the text cursor to the desired location, change its color to white, and print the "Howdy" text on the screen. By post-obit the same procedure, we display the red YouTube text as well.
//Draw white frame tft.drawRect(0,0,319,240,WHITE); //Impress "Howdy" Text tft.setCursor(100,thirty); tft.setTextColor(WHITE); tft.setTextSize(4); tft.print("Hello"); //Impress "YouTube!" text tft.setCursor(80,100); tft.setTextColor(Scarlet); tft.setTextSize(4); tft.print("YouTube!");
Next, we create the ruby Youtube subscribe button. To achieve this, nosotros create a cherry-red rectangle and for our size requirements, we gear up the x and y pixel coordinates of the elevation left corner point of this rectangle to (60,180), define its width as 200 pixels, its elevation every bit 40 pixels and set pixel coordinates for the bottom right of the rectangle to (260, 220). To make the rectangle look more like a button, we draw a similar white rectangle outside of the cherry-red rectangle and write the "Subscribe" text on it.
//Create Red Button tft.fillRect(60,180, 200, 40, Ruby-red); tft.drawRect(60,180,200,40,WHITE); tft.setCursor(80,188); tft.setTextColor(WHITE); tft.setTextSize(3); tft.print("Subscribe!");
With the setup function all done, we move to the loop function, the algorithm in functioning for the loop section is elementary, each time the user clicks on the screen, we convert the point coordinates of the touch on point into pixels using the Map function. Afterwards conversion, if that signal is inside the red rectangle expanse, it means that the user has pressed the button, so we disable the button by setting this variable to false and we clear the screen so to display the "Thank you for Subscribing" bulletin on the screen.
void loop() { TSPoint p = ts.getPoint(); //Get touch point if (p.z > ts.pressureThreshhold) { Series.print("X = "); Serial.print(p.x); Serial.print("\tY = "); Series.impress(p.y); Serial.impress("\n"); p.x = map(p.x, TS_MAXX, TS_MINX, 0, 320); p.y = map(p.y, TS_MAXY, TS_MINY, 0, 240); if(p.x>60 && p.x<260 && p.y>180 && p.y<220 && buttonEnabled) // The user has pressed inside the scarlet rectangle { buttonEnabled = faux; //Disable push //This is important, because the libraries are sharing pins pinMode(XM, OUTPUT); pinMode(YP, OUTPUT); //Erase the screen tft.fillScreen(BLACK); //Draw frame tft.drawRect(0,0,319,240,WHITE); tft.setCursor(50,50); tft.setTextColor(WHITE); tft.setTextSize(3); tft.impress("Give thanks you for\north\northward subscribing!"); } delay(x); } }
The complete code for this project is beneath and also attached under the download section.
////////////////////////////////////////////// // 2.8" TOUCH SCREEN DEMO // // // // http://world wide web.educ8s.tv // ///////////////////////////////////////////// #include <Adafruit_TFTLCD.h> #include <Adafruit_GFX.h> #include <TouchScreen.h> #define LCD_CS A3 #define LCD_CD A2 #define LCD_WR A1 #ascertain LCD_RD A0 #ascertain LCD_RESET A4 #define TS_MINX 204 #define TS_MINY 195 #define TS_MAXX 948 #define TS_MAXY 910 #define YP A2 // must be an analog pin, employ "An" notation! #define XM A3 // must exist an analog pin, apply "An" notation! #ascertain YM viii // can be a digital pin #define XP nine // can exist a digital pin #ascertain BLACK 0x0000 #define BLUE 0x001F #define RED 0xF800 #ascertain GREEN 0x07E0 #define CYAN 0x07FF #define MAGENTA 0xF81F #define Yellow 0xFFE0 #ascertain WHITE 0xFFFF Adafruit_TFTLCD tft(LCD_CS, LCD_CD, LCD_WR, LCD_RD, LCD_RESET); TouchScreen ts = TouchScreen(XP, YP, XM, YM, 300); boolean buttonEnabled = truthful; void setup() { Serial.brainstorm(9600); Serial.print("Starting..."); tft.reset(); tft.brainstorm(0x9325); tft.setRotation(1); tft.fillScreen(Blackness); //Describe white frame tft.drawRect(0,0,319,240,WHITE); //Print "Howdy" Text tft.setCursor(100,30); tft.setTextColor(WHITE); tft.setTextSize(4); tft.impress("Hello"); //Print "YouTube!" text tft.setCursor(80,100); tft.setTextColor(RED); tft.setTextSize(4); tft.impress("YouTube!"); //Create Red Button tft.fillRect(60,180, 200, 40, RED); tft.drawRect(60,180,200,40,WHITE); tft.setCursor(fourscore,188); tft.setTextColor(WHITE); tft.setTextSize(three); tft.impress("Subscribe!"); } void loop() { TSPoint p = ts.getPoint(); //Go touch point if (p.z > ts.pressureThreshhold) { Series.print("X = "); Serial.print(p.10); Serial.impress("\tY = "); Series.print(p.y); Serial.print("\northward"); p.x = map(p.x, TS_MAXX, TS_MINX, 0, 320); p.y = map(p.y, TS_MAXY, TS_MINY, 0, 240); if(p.10>60 && p.x<260 && p.y>180 && p.y<220 && buttonEnabled)// The user has pressed within the blood-red rectangle { buttonEnabled = simulated; //Disable button //This is important, because the libraries are sharing pins pinMode(XM, OUTPUT); pinMode(YP, OUTPUT); //Erase the screen tft.fillScreen(Black); //Draw frame tft.drawRect(0,0,319,240,WHITE); tft.setCursor(fifty,50); tft.setTextColor(WHITE); tft.setTextSize(iii); tft.print("Thank you for\n\n subscribing!"); } delay(10); } }
Demo
Copy the code above and create a new Arduino sketct. Ensure the libraries are installed and upload the code to the setup described under the schematics department. One time the upload is complete, you should meet the display come up equally shown below.
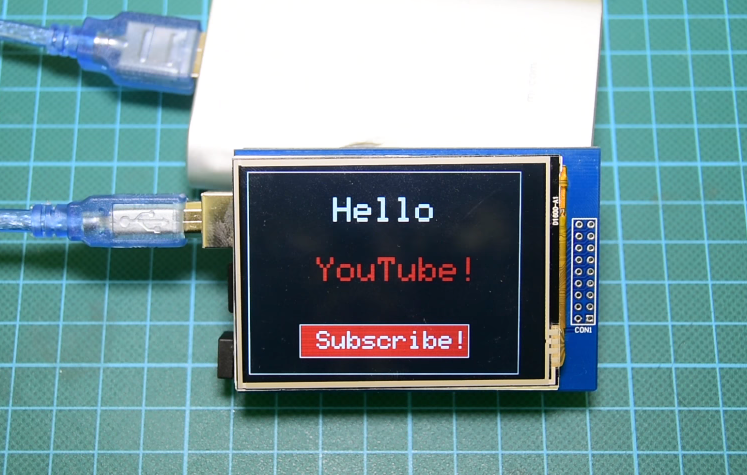
Every bit soon every bit the subscribe button is pressed, the screen below is displayed.
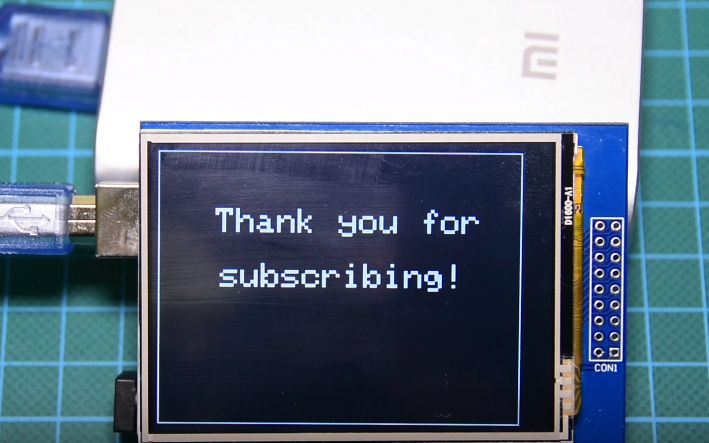
That'due south it for this tutorial guys. Experience free to aggrandize this code for utilise in your projects and practice not hesitate to allow me know via the comment department if you have any questions.
Till Next time.
The video version of this tutorial is on youtube.
Source: https://www.electronics-lab.com/project/using-the-geekcreit-ili9325-2-8-touchscreen-display-with-arduino/
Posted by: murphyussighboult1999.blogspot.com
This is my first time visit to your blog and I am very interested in the articles that you serve. Provide enough knowledge for me. Thank you for sharing useful and don't forget, keep sharing useful info: https://www.yichuangscreen.com/product/detail/75-inch-large-interactive-touch-screen-smart-board.html
ReplyDelete